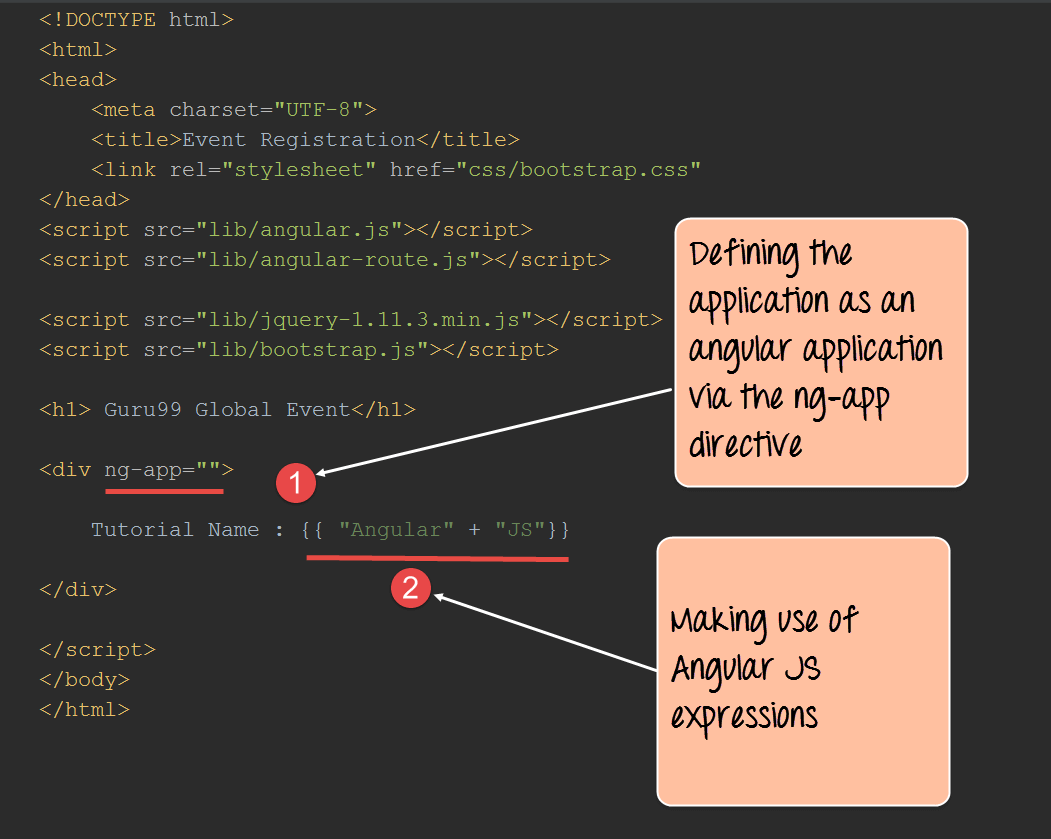
Directives are a way to teach HTML new tricks. During DOM compilation directives are matched against the HTML and executed. This allows directives to register behavior, or transform the DOM. Angular comes with a built in set of directives which are useful for building web applications but can be extended such that HTML can be turned into a declarative domain specific language (DSL).
Invoking Directives from HTML:
Directives have camel cased names such as ngBind. The directive can be invoked by translating the camel case name into snake case with these special characters :, -, or _. Optionally the directive can be prefixed with x-, or data- to make it HTML validator compliant. Here is a list of some of the possible directive names: ng:bind, ng-bind, ng_bind, x-ng-bind and data-ng-bind.
The directives can be placed in element names, attributes, class names, as well as comments. Here are some equivalent examples of invoking myDir. (However, most directives are restricted to attribute only.)
<span my-dir=="exp"></span>
<span class=="my-dir: exp;"></span>
<my-dir></my-dir>
<!-- directive: my-dir exp -->
Directives can be invoked in many different ways, but are equivalent in the end result as shown in the following example
Index.html:
<!doctype html>
<html ng-app>>
<head>
<script src=="http://code.angularjs.org/1.0.4angular.min.js"></script>
<script src=="script.js"></script>
</head>
<body>
<div ng-controller=="Ctrl1">>
Hello <input ng-model=='name'>> <hr/>
<span ng:bind="name"> <span ng:bind=="name"></span> <br/>
<span ng_bind="name"> <span ng_bind=="name"></span> <br/>
<span ng-bind="name"> <span ng-bind=="name"></span> <br/>
<span data-ng-bind="name"> <span data-ng-bind=="name"></span> <br/>
<span x-ng-bind="name"> <span x-ng-bind=="name"></span> <br/>
</div>
</body>
</html>
Script.Js:
function Ctrl1(($scope)) {{
$scope..name == 'angular';;
}
End test:
it('should show off bindings',, function() {
expect(element('div[ng-controller="Ctrl1"] span[ng-bind]').text()).toBe('angular');
});
String Interpolation:
During the compilation process the compiler matches text and attributes using the $interpolate service to see if they contain embedded expressions. These expressions are registered as watches and will update as part of normal digest cycle.
<a href=="img/{{username}}.jpg">>Hello {{username}}!</a>
Compilation Process and Directive Matching:
Compilation of HTML happens in three phases:
First the HTML is parsed into DOM using the standard browser API. This is important to realize because the templates must be parsable HTML. This is in contrast to most templating systems that operate on strings, rather than on DOM elements.
The compilation of the DOM is performed by the call to the $compile() method. The method traverses the DOM and matches the directives. If a match is found it is added to the list of directives associated with the given DOM element. Once all directives for a given DOM element have been identified they are sorted by priority and their compile() functions are executed.
The directive compile function has a chance to modify the DOM structure and is responsible for producing a link() function explained next. The $compile() method returns a combined linking function, which is a collection of all of the linking functions returned from the individual directive compile functions.
Link the template with scope by calling the linking function returned from the previous step. This in turn will call the linking function of the individual directives allowing them to register any listeners on the elements and set up any watches with the scope. The result of this is a live binding between the scope and the DOM. A change in the scope is reflected in the DOM.
. var $compile == ...;
var scope == ...;
var html == '<div ng-bind='exp'></div>';;
var template == angular..element((html);
var linkFn == $compile((template);
linkFn((scope);
Reasons behind the compile/link separation:
At this point you may wonder why the compile process is broken down to a compile and link phase. To understand this, let's look at a real world example with a repeater:
Hello {{user}}, you have these actions::
<ul>
<<li ng--repeat=="action in user.actions">>
{{action..description}}
<</li>
</ul>
The short answer is that compile and link separation is needed any time a change in model causes a change in DOM structure such as in repeaters. When the above example is compiled, the compiler visits every node and looks for directives. The {{user}} is an example of an interpolation directive. ngRepeat is another directive. But ngRepeat has a dilemma. It needs to be able to quickly stamp out new lis for every action in user.actions.
This means that it needs to save a clean copy of the li element for cloning purposes and as new actions are inserted, the template li element needs to be cloned and inserted into ul. But cloning the li element is not enough. It also needs to compile the li so that its directives such as {{action.descriptions}} evaluate against the right scope. A naive method would be to simply insert a copy of the li element and then compile it. But compiling on every li element clone would be slow, since the compilation requires that we traverse the DOM tree and look for directives and execute them.
If we put the compilation inside a repeater which needs to unroll 100 items we would quickly run into performance problems. The solution is to break the compilation process into two phases; the compile phase where all of the directives are identified and sorted by priority, and a linking phase where any work which links a specific instance of the scope and the specific instance of an li is performed. ngRepeat works by preventing the compilation process from descending into the li element.
Instead the ngRepeat directive compiles li separately. The result of the li element compilation is a linking function which contains all of the directives contained in the li element, ready to be attached to a specific clone of the li element. At runtime the ngRepeat watches the expression and as items are added to the array it clones the li element, creates a new scope for the cloned li element and calls the link function on the cloned li.
For more in-depth knowledge on Angular enroll for a live free demo on angularjs online training with 24x7 Guidance support and lifetime Access