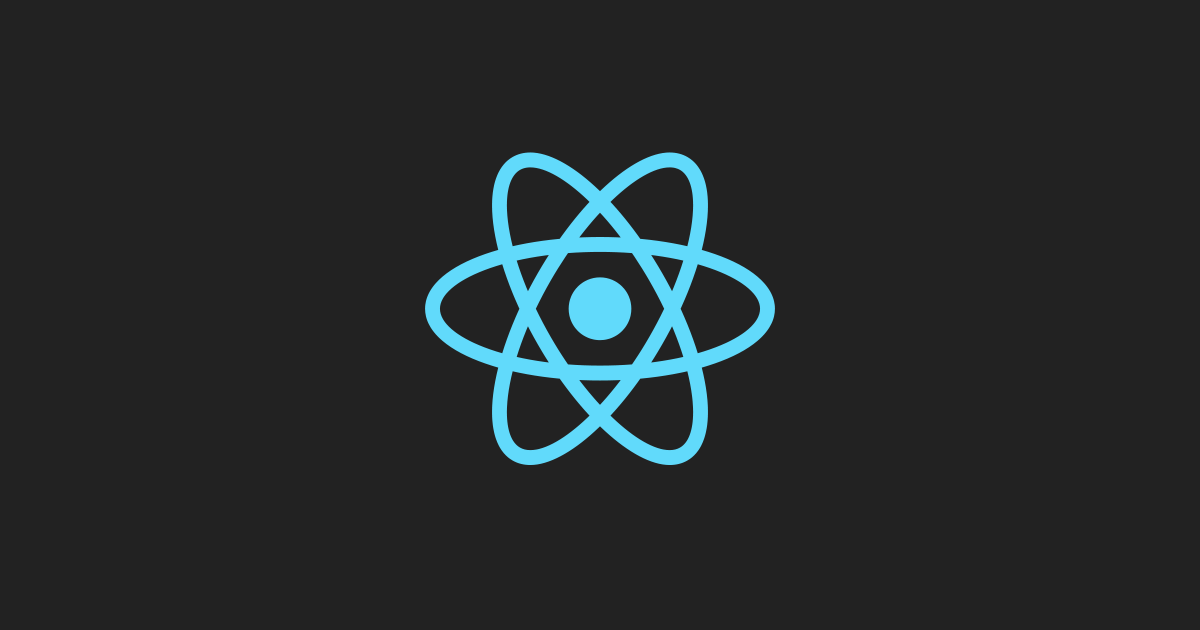
What is code splitting in React js?
Code Splitting in react js simply spilt your bundle generated by Wepack or Browserify into multiple and load them dynamically.
Why splitting is important: We develop react app. Our code imports many third-party libraries. Bundle generated by web pack or Browserify also gets fatter and fatter. This fat budle.js file takes time to get downloaded in the browser and user feel the uneasiness with the web application.
Advantages: Though you have not reduced code writing you have reduced the code needed to be downloaded. This lazy load can improve the performance of your web application dramatically.
Ways to implement Code-Splitting:
- import as a function:
// math.js export function add(a, b) { return a + b; }
import("./math").then(math => { console.log(math.add(16, 26)); });
When Webpack comes across this syntax, it automatically starts code-splitting your app. If you’re using Create React App, this is already configured for you and you can start using it immediately.
If you’re setting up Webpack yourself, you’ll probably want to read the Web packs guide on code splitting. Your Webpack config should look vaguely like this.
- React Loadable Library: React Loadable wraps dynamic imports in a nice, React-friendly API for introducing code splitting into your app at a given component.
//without code splitting import OtherComponent from './OtherComponent'; const MyComponent = () => ( <OtherComponent/> );
//with code splitiing import Loadable from 'react-loadable'; const LoadableOtherComponent = Loadable({ loader: () => import('./OtherComponent'), loading: () => <div>Loading...</div>, }); const MyComponent = () => ( <LoadableOtherComponent/> );
React Loadable helps you create loading states, error states, timeouts, preloading, and more. It can even help you server-side render an app with lots of code-splitting.
- Code Splitting in React Router: Though an Idea of splitting the code seems awesome, where to split the code is a tricky decision. The route is the best place to lazy load the things.
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom'; import Loadable from 'react-loadable'; const Loading = () => <div>Loading...</div>; const Home = Loadable({ loader: () => import('./routes/Home'), loading: Loading, }); const About = Loadable({ loader: () => import('./routes/About'), loading: Loading, }); const App = () => ( <Router> <Switch> <Route exact path="/" component={Home}/> <Route path="/about" component={About}/> </Switch> </Router> );
Looking for ReactJS Development Company, hire our dedicated developers!