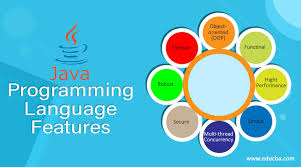
Introduction:
In the world of Java development, the concept of objects plays a central role. Objects are the fundamental building blocks of Java programs, representing entities or concepts in the real world. Creating objects in Java involves several key steps, from declaring a class to instantiating an object. This article will provide a comprehensive guide to creating objects in Java, exploring the necessary syntax and best practices. Whether you are a seasoned Java developer or aspiring to start a career in software development, understanding object creation is essential for building robust and efficient Java applications.
Understanding Objects:
Before diving into the process of creating objects, it's important to grasp the concept of objects in Java. In object-oriented programming, objects are instances of classes, which serve as blueprints for creating objects. A class defines the properties (variables) and behaviors (methods) that objects of that class will possess. Objects can be considered containers that encapsulate data and functionality, allowing for modular and reusable code.
The syntax for Object Creation:
In Java, object creation involves two primary steps: declaring a class and instantiating an object. Let's explore each step in detail:
- Declaring a Class:
- To create an object, you first need to define a class. A class declaration consists of a class name, member variables, and methods. Here's an example:
java Copy code public class MyClass { // Member variables private int myVariable; // Methods public void method() { // Method body } }
- In this example, the class "MyClass" is declared with a private member variable "myVariable" and a public method "myMethod". This class serves as a blueprint for creating objects.
- Instantiating an Object:
- Once you have defined a class, you can create objects of that class using the "new" keyword. Here's an example:
java Copy code MyClass myObject = new MyClass();
- In this line of code, "myObject" is an object of the class "MyClass" created using the "new" keyword. This process is called instantiation. The "new" keyword allocates memory for the object and invokes the class's constructor to initialize the object.
Best Practices for Object Creation:
To ensure efficient and maintainable code, consider the following best practices when creating objects in Java:
- Encapsulation:
- Encapsulate data within objects by making member variables private and providing access through methods (getters and setters). This promotes data integrity and abstraction.
- Constructor Overloading:
- Use constructor overloading to provide different ways to initialize objects. This allows flexibility and customization when creating objects.
- Singleton Pattern:
- Implement the Singleton pattern when you need to ensure that only one instance of a class exists. This can be useful for managing shared resources or maintaining global state.
- Factory Design Pattern:
- Utilize the Factory design pattern to encapsulate object creation logic. Factories provide a centralized way of creating objects, allowing for flexibility and code decoupling.
Conclusion:
Creating objects is a fundamental aspect of Java development. By understanding the syntax and best practices for object creation, you can build robust and efficient Java applications. Whether you are pursuing a career in software development or looking to enhance your Java skills, mastering object creation is essential. Keep exploring and practicing, and you'll become proficient in creating objects that power your Java programs.
Remember, JAVA Development and a career in Software Development offer numerous opportunities for Java developers. By acquiring expertise in object creation and other essential Java concepts, you can unlock a world of Java developer jobs and build a successful career in this dynamic field.
Contact Us:
If you are interested in learning more about JAVA Development or pursuing a career in Software Development, contact us at [Contact Information]. Our expert team is ready to assist you with your educational and career goals.
Keywords: JAVA Development, a career in Software development, Java developer jobs, creating objects in Java, object-oriented programming, object creation, Java classes, object instantiation, best practices in Java, encapsulation, constructor overloading, Singleton pattern, Factory design pattern.