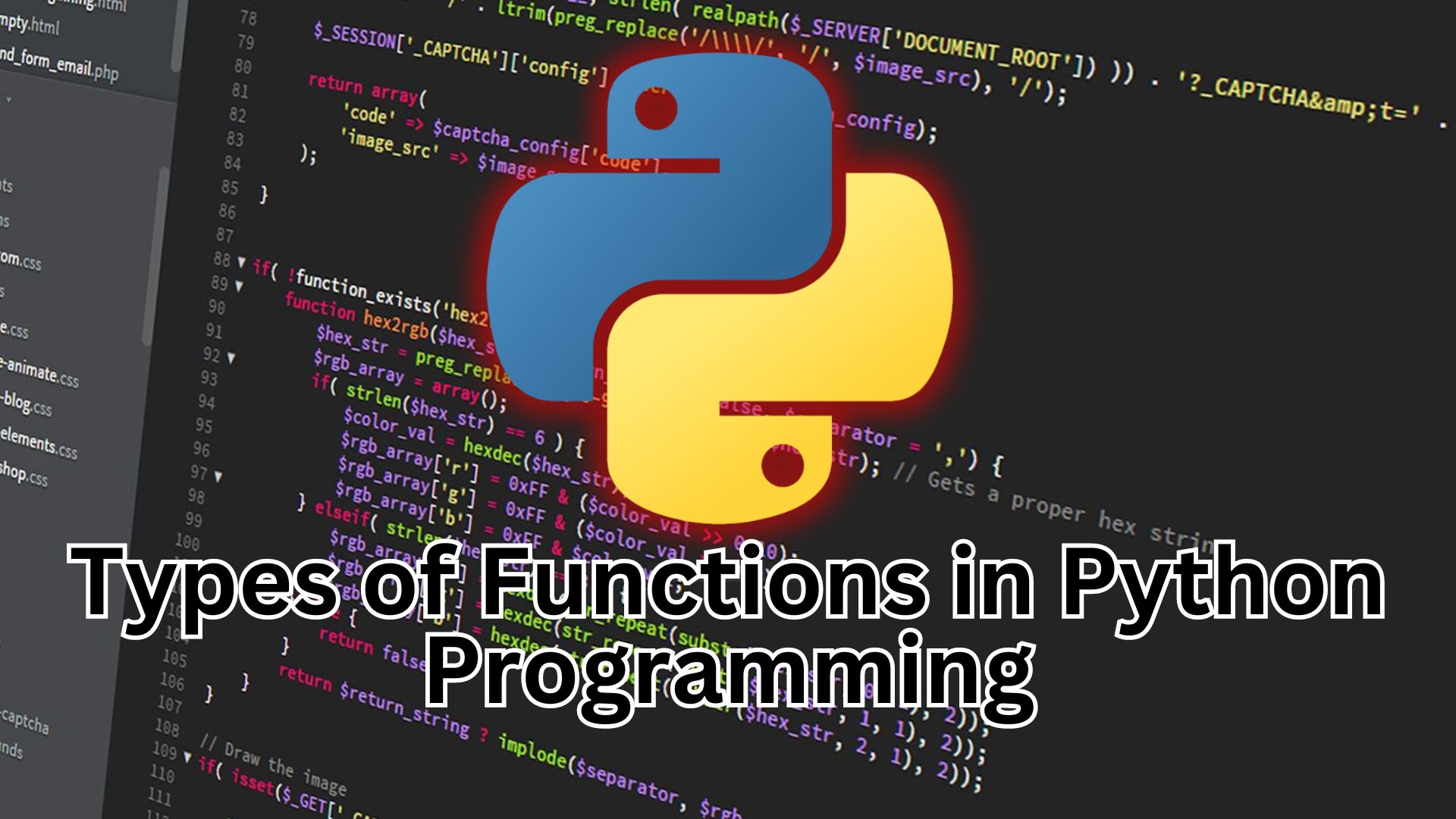
Functions in Python are blocks of organized, reusable code that perform a specific task. They help in modularizing code, making it more readable, maintainable, and efficient. Python offers various types of functions, each serving different purposes and catering to diverse programming needs. Understanding these types is crucial for harnessing the full power of Python's functional capabilities. In this comprehensive guide, we will explore the different types of functions in Python and their applications.
1. Built-in Functions:
- Built-in functions are those functions that are readily available in Python, and you can use them directly without any prior definition.
- Examples include `print()`, `len()`, `input()`, `range()`, `sum()`, etc.
- These functions provide essential functionalities for common tasks like input/output operations, data manipulation, iteration, etc.
- They serve as the foundation for many Python programs, offering convenience and efficiency.
2. User-defined Functions:
- User-defined functions are created by the programmer to perform specific tasks as per requirements.
- They allow encapsulating reusable code into a single function block, promoting code reusability and maintainability.
- To define a user-defined function, you use the `def` keyword followed by the function name and parameters.
- Example:
```python
def greet(name):
print("Hello, " + name + "!")
```
3. Anonymous Functions (Lambda Functions):
- Lambda functions are small, anonymous functions defined using the `lambda` keyword.
- They can have any number of parameters but only one expression.
- Lambda functions are typically used when you need a simple function for a short period and don't want to define a full-fledged function using `def`.
- Example:
```python
square = lambda x: x * x
```
4. Recursive Functions:
- Recursive functions are functions that call themselves in order to solve a problem.
- They are particularly useful for solving problems that can be broken down into smaller, similar sub-problems.
- Examples of recursive functions include factorial calculation, Fibonacci sequence generation, etc.
- Example (factorial calculation):
```python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
```
5. Higher-order Functions:
- Higher-order functions are functions that can take other functions as arguments or return functions as results.
- They enable functional programming paradigms like map, filter, reduce, etc.
- Example (map function):
```python
numbers = [1, 2, 3, 4, 5]
squared = list(map(lambda x: x * x, numbers))
```
6. Generator Functions:
- Generator functions are used to create iterators, i.e., a sequence of values that can be iterated over.
- They use the `yield` keyword to return data one element at a time, pausing execution between each yield.
- Generator functions are memory efficient as they produce values on-the-fly rather than storing them in memory.
- Example:
```python
def countdown(n):
while n > 0:
yield n
n -= 1
```
7. Decorator Functions:
- Decorator functions are used to modify the behavior of other functions or methods.
- They allow adding functionality to existing functions without modifying their structure.
- Decorators are implemented using the `@decorator_name` syntax.
- Example:
```python
def uppercase_decorator(func):
def wrapper(text):
result = func(text)
return result.upper()
return wrapper
@uppercase_decorator
def greet(name):
return "Hello, " + name + "!"
```
Conclusion:
Functions are an integral part of Python programming, enabling code organization, reuse, and abstraction. Understanding the different types of functions in Python is essential for writing efficient, maintainable, and scalable code. Whether you're working with built-in functions, defining your own functions, or leveraging advanced concepts like lambda functions, recursion, or decorators, mastering the use of functions will greatly enhance your proficiency as a Python programmer.