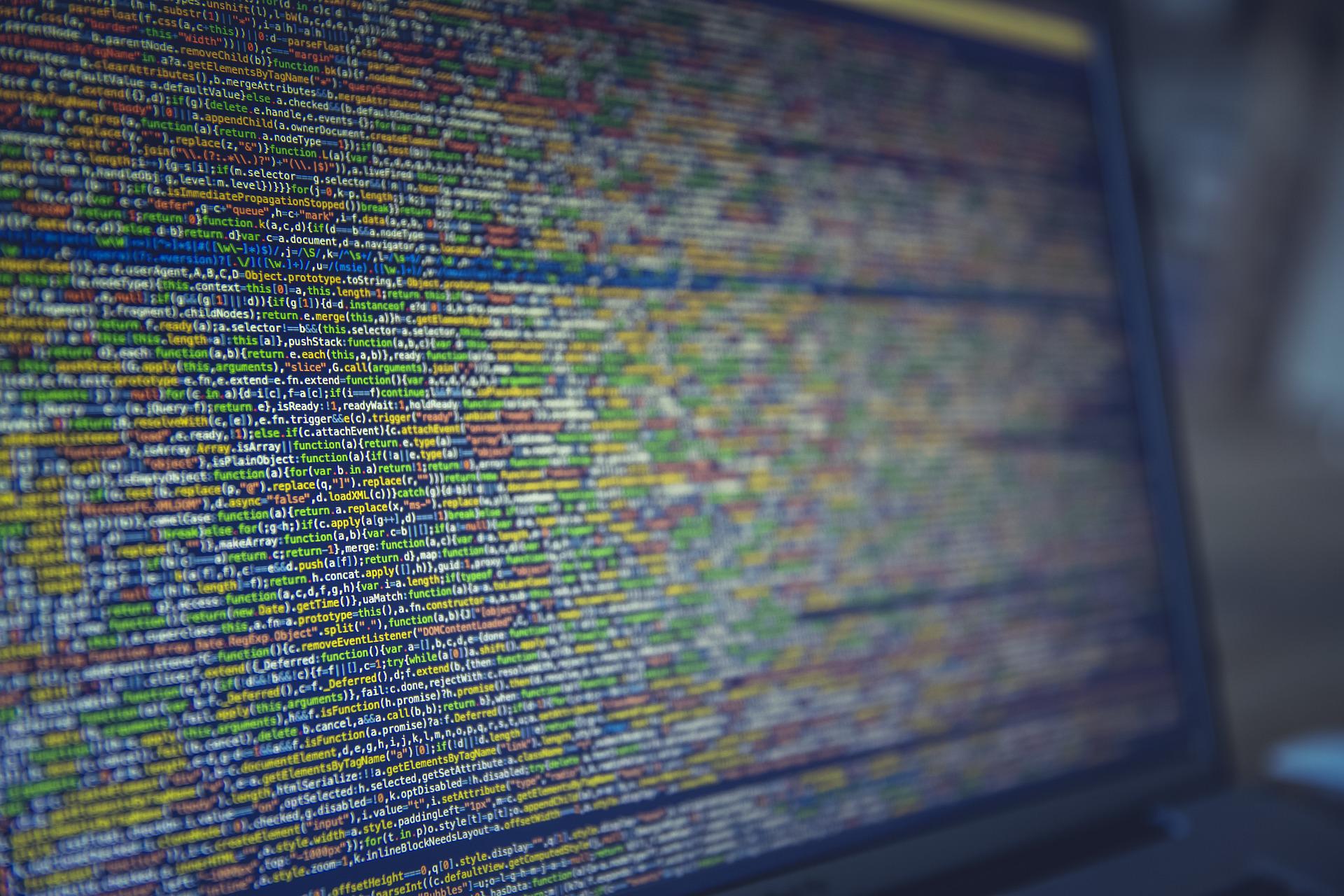
If you are into programming, you must have encountered the term "Algorithm." Algorithms are widely used in programming and many other streams of computer science.
But what exactly is the definition of an algorithm? So, in today's blog, you will learn all about algorithms and their importance in computer science and technology.
Moreover, you will also learn about some of the widely used algorithms in programming.
What is an Algorithm?
Starting with the definition, an algorithm can be defined as a set of steps, rules, and instructions following which one can solve a specific problem. It means that an algorithm is a way of solving problems in computer science.
Moreover, it is also necessary to understand that an algorithm is specific to solving a particular problem. It is also possible that you will be using multiple algorithms to solve a problem, as the problem contains multiple problems solvable by algorithms.
Since algorithms are used to solve problems and problems are not bound to a single subject, many subjects contain algorithms. You may also get introduced to algorithms in mathematics.
In mathematics, there are a lot of problems based on algorithms. Moreover, computer science, operations research, data science, and Artificial intelligence are also subjects where algorithms are important.
In data science, numerous algorithms help process and analyze a large amount of data for industries like healthcare, marketing, finance, etc.
Similarly, the current date AI technology is based on algorithms. These algorithms help the AI to gather data, process data, recognize images and other media, etc.
Moreover, it also helps AI to keep learning and training by itself. However, AI and Data Science are relatively newer than computer science. So there's no doubt that computers have used algorithms for a very long time.
So let's learn about two very useful programming algorithms, namely, Kadane's Algorithm and bubble sort algorithm.
Kadane's Algorithm
Kadane's algorithm is a popular algorithm in programming. It is used to find the maximum subarray sum in a given array of numbers. It is an efficient algorithm with a time complexity of O(n), where n is the size of the array. This algorithm is named after its inventor, Jay Kadane.
The problem of finding the maximum subarray sum is as follows: Given an array of integers, we need to find the contiguous subarray (a subarray with consecutive elements) with the largest sum. The subarray can be empty, meaning it contains no elements.
Kadane's algorithm is based on the idea of dynamic programming. It iterates through the array, keeping track of the maximum sum encountered so far and the maximum sum ending at each position.
At each step, it considers whether extending the current subarray or starting a new subarray would result in a larger sum.
For example, let an array of numbers {1, 2, 3, 4, 5}. Now there can be multiple sub-arrays possible from this array.
subarrays of {1, 2, 3, 4, 5} = [{1}, {2}, {3}, {4}, {5}, {1, 2}, {1, 2, 3}.....]
So, to find out the subarray with the maximum sun, it is necessary to get the sum of all these subarrays individually and compare all to get the largest. But it is more interesting and easy to see how Kadane's algorithm solves this rather than doing it manually.
So two important variables are getting used. The first is "currentSum()," which calculates the sum of individual aub arrays. Moreover, the second variable is "maxSum()."
This variable keeps track of the program's highest sum until now. So, throughout the process, the 'maxSum()' gets updated. Moreover, when the process gets complete, the latest "maxSum()" sub-array is the answer.
Here is the program for Kadane's algorithm in C solving maximum subarray problem for {-2, 1, -3, 4, -1, 2, 1, -5, 4}
Input
Output
Bubble Sort Algorithm
Bubble sort is a commonly used and straightforward sorting algorithm that compares neighboring elements in a list and exchanges them if they are not in the desired order.
It is named "bubble sort" because smaller elements gradually "bubble" to the beginning of the array as the algorithm progresses.
The fundamental concept underlying bubble sort involves traversing the array multiple times, examining neighboring elements, and swapping them if necessary.
The process continues until the array gets sorted. The algorithm repeats this process for each element in the array, gradually moving larger elements toward the end.
Let's understand it with an example. Here is an array which is as follows: {7, 2, 13, 1, 5, 8}. Now, if you are asked to sort this array in ascending order, you start with placing the smallest number in the first place and then placing numbers in ascending numbers.
But an algorithm can't be fed that way as this way of solving includes a lot of intuition. Moreover, this intuition results in randomness. So, the bubble sort algorithm uses a different approach.
Here is a step-by-step breakdown of the bubble sort algorithm:
- Start with an unsorted array of elements.
- Compare the first element with the second element. If the first one is greater than the second, swap them.
- Move to the next pair of elements (second and third) and compare them. Again, swap if necessary.
- Continue this process, comparing and swapping adjacent elements until you reach the end of the array.
- At the end of the first pass, the most significant element will have "bubbled" to the end of the array.
- Repeat steps 2-5 for the remaining elements, excluding the last element of the previous pass, as it is already in its correct position.
- Continue passing through the array until no more swaps are needed. This indicates that the array is fully sorted in ascending order.
Here is a simple implementation of bubble sort in Python for an array [64, 34, 25, 12, 22, 11, 90]:
Input
In the above code, the `bubble_sort` function takes an array as input and performs the bubble sort algorithm on it. The nested `for` loops iterate through the array, comparing adjacent elements and swapping them if necessary. After the sorting is complete, the sorted array is printed.
Output
Wrapping Up
Algorithms are everywhere, from mathematics to computer science. However, using algorithms in programming is necessary to make it simple to create powerful programs.
The above-listed two algorithms are among the most simple and easy-to-understand algorithms. So, it will benefit you to start learning algorithms from these two algorithms.